Secure Coding in C and C++
Secure Coding in C and C++ is a two day training course that provides a detailed explanation of common programming errors in C and C++ and describes how these errors can lead to code that is vulnerable to exploitation.
This course concentrates on security issues intrinsic to the C and C++ programming languages and associated libraries. You will learn to identify common security flaws including:
- Memory safety
- Insecure Integer operations
- Undefined behaviors
For each of these security flaws, we demonstrate specific remediation techniques as well as general secure coding practices that help prevent the introduction of vulnerabilities.
Material in this presentation was derived from the Addison-Wesley books Secure Coding in C and C++ and The CERT C Secure Coding Standard.
Agenda
Day 1: Strings
- Introduction
- Standards
- Conformance
- Behaviors
- Errors
- Common errors using NTBS
- improperly bounded string copies
- null-termination errors
- truncation
- write outside array bounds
- off-by-one errors
- improper data sanitization
- Common errors using basic_string
- String Vulnerabilities
- Program Stack
- Buffer Overflow
- Code Injection
- Arc Injection
- Exercise: Identify String Problems
- Mitigation Strategies
- Prevention Strategies
- Detection Strategies
- Summary
Day 2: Integers
- Integer Types
- Integer Data Types
- Unsigned integer types
- Wraparound
- Signed integer types
- Signed integer ranges
- Overflow
- Character types
- Other integer types
- Integer Conversions
- Integer conversion rank
- Integer promotions
- Usual arithmetic conversions
- Conversions to unsigned integer types
- Conversions to signed integer types
- Conversion implications
- Integer Operations
- Addition
- Multiplication
- Division/remainder
- Right shift
- Exercise: Reviewing Code for Integer Defects
- Integer Vulnerabilities
- Wrap around
- Conversion error
- Truncation
- Non-exceptional
- Mitigation Strategies
- Integer type selection
- Safe integer operations
- Compiler Strategies
- Testing and reviews
- Summary
- Integer Data Types
- Dangerous Optimizations
- Compiler Optimizations
- Constant Folding
- Adding a Pointer and an Integer
- Integer Overflow
- GCC Options
- Volatile
- Strict Aliasing
- Optimization Suggestions
- Null pointer
- Uninitialized Reads
- C11 Analyzability Annex
- Summary and Recommendations
Who should attend
Secure Coding in C and C++ should be useful to anyone involved in the development or maintenance of software in C and C++.
- If you are a C or C++ programmer, this book will teach you how to identify common programming errors that result in software vulnerabilities, understand how these errors are exploited, and implement a solution in a secure fashion.
- If you are a software project manager, this book identifies the risks and consequences of software vulnerabilities to guide investments in developing secure software.
- If you are a computer science student, this book will teach you programming practices that will help you to avoid developing bad habits and enable you to develop secure programs during your professional career.
- If you are a security analyst, this book provides a detailed description of common vulnerabilities, identifies ways to detect these vulnerabilities, and offers practical avoidance strategies.
Pre-requisites
The course assumes basic C and C++ programming skills, but does not assume an in-depth knowledge of software security. The ideas presented apply to various development environments, but the examples are specific to Microsoft Visual Studio and Linux/GCC and the Intel Architecture.
Software to install
Students are also encouraged to bring their own C and C++ programming language development environments (compiler, editor, etc.), such as Microsoft Visual Studio, Xcode, GCC, or Clang.
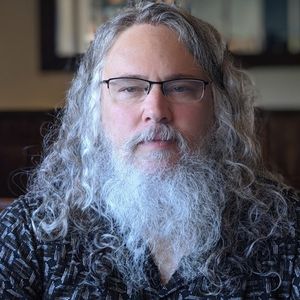
Robert C. Seacord is the Standardization Lead at Woven by Toyota where he works on the Software Craft. Robert is convener of the ISO/IEC JTC1/SC22/WG14 international standardization working group for the C programming language. He is the author of seven books, including Effective C, Second Edition (No Starch Press, 2024), The CERT C Coding Standard, Second Edition (Addison-Wesley, 2014) Secure Coding in C and C++, Second Edition (Addison-Wesley, 2013), and Java Coding Guidelines: 75 Recommendations for Reliable and Secure Programs (Addison-Wesley, 2014). He has also published more than 50 papers on software security, component-based software engineering, Web-based system design, legacy-system modernization, component repositories and search engines, and user interface design and development. Robert has been teaching secure coding in C and C++ to private industry, academia, and government since 2005. He started programming professionally for IBM in 1982.