Secure Coding in C and C++
Secure Coding in C and C++ is a two day training course that provides a detailed explanation of common programming errors in C and C++ and describes how these errors can lead to vulnerable software.
This course concentrates on security issues intrinsic to the C and C++
programming languages and associated libraries. You will learn to identify
vulnerabilities ensuing from common security flaws including:
- Insecure Integer operations
- Undefined behaviors
- Memory safety
This course covers specific remediation techniques for each category of
security flaws as well as general secure coding practices that help
prevent the introduction of vulnerabilities.
Material in this presentation was derived from the Addison-Wesley books
Secure Coding in C and C++ and The CERT C Secure Coding Standard.
Agenda
Day 1: Integers
- Integer Types
- Integer Data Types
- Unsigned integer types
- Wraparound
- Signed integer types
- Signed integer ranges
- Overflow
- Character types
- Other integer types
- Integer Conversions
- Integer conversion rank
- Integer promotions
- Usual arithmetic conversions
- Conversions to unsigned integer types
- Conversions to signed integer types
- Conversion implications
- Integer Operations
- Addition
- Multiplication
- Division/remainder
- Right shift
- Exercise: Reviewing Code for Integer Defects
- Integer Vulnerabilities
- Wrap around
- Conversion error
- Truncation
- Non-exceptional
- Mitigation Strategies
- Integer type selection
- Safe integer operations
- Compiler Strategies
- Testing and reviews
- Summary
Day 2: Dangerous Optimizations & Dynamic Memory
- Compiler Optimizations
- Constant Folding
- Adding a Pointer and an Integer
- Integer Overflow
- GCC Options
- Volatile
- Strict Aliasing
- Optimization Suggestions
- Null pointer
- Uninitialized Reads
- C11 Analyzability Annex
- Summary and Recommendations
Dynamic Memory
- Commo Dynamic Memory Management Errors
- Doug Lea's Memory Allocator
- Buffer Overflows
- Double-free
- Mitigation strategies
- Exercise: Finding memory errors
Who should attend
Secure Coding in C and C++ is useful to anyone involved in the development
or maintenance of software in C and C++. If you are a:
- C or C++ programmer, this book will teach you how to identify common
programming errors that result in software vulnerabilities, understand
how these errors are exploited, and implement a solution in a secure
fashion. - Software project manager, this book identifies the risks and
consequences of software vulnerabilities to guide investments in
developing secure software. - Computer science student, this book will teach you programming
practices that will help you to avoid developing bad habits and enable
you to develop secure programs during your professional career. - Security analyst, this book provides a detailed description of common
vulnerabilities, identifies ways to detect these vulnerabilities, and
offers practical avoidance strategies.
Pre-requisites
The course assumes basic C and C++ programming skills, but does not assume
an in-depth knowledge of software security. The ideas presented apply to
various development environments, but the examples are specific to
Microsoft Visual Studio and Linux/GCC and the Intel Architecture.
Software to install
Students are also encouraged to bring their own C and C++ programming
language development environments (compiler, editor, etc.), such as
Microsoft Visual Studio, Xcode, GCC, or Clang.
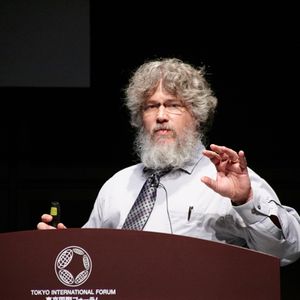
Robert C. Seacord is the Standardization Lead at Woven by Toyota he works on the Software Craft. Robert was previously a Technical Director at NCC Group, Secure Coding Manager at Carnegie Mellon's Software Engineering Institute, and an adjunct professor in the School of Computer Science and the Information Networking Institute at Carnegie Mellon University.
He is the author of seven books, including Effective C: An Introduction to Professional C Programming (No Starch Press, 2020), The CERT C Coding Standard, Second Edition (Addison-Wesley, 2014) Secure Coding in C and C++, Second Edition (Addison-Wesley, 2013), and Java Coding Guidelines: 75 Recommendations for Reliable and Secure Programs (Addison-Wesley, 2014). He has also published more than 50 papers on software security, component-based software engineering, Web-based system design, legacy-system modernization, component repositories and search engines, and user interface design and development. Robert has been teaching secure coding in C and C++ to private industry, academia, and government since 2005. He started programming professionally for IBM in 1982, working in communications and operating system software, processor development, and software engineering and also has worked at the X Consortium, where he developed and maintained code for the Common Desktop Environment and the X Window System. Robert is on the Advisory Board for the Linux Foundation is an expert at the ISO/IEC JTC1/SC22/WG14 international standardization working group for the C programming language.